17. Turtle
Turtle
is part of the Python API used to draw graphics on the screen. The main objects in Turtle are the Screen
and Pen
. The Screen is the canvas that is being drawn onto and the Pen is the object that you use to draw. As always, the best way to learn Turtle is to see some examples and start coding your own creations.
17.1. Draw a line
Here’s a simple program to draw a square.
1import turtle
2
3# w is an instance of Screen
4# we choose w as the variable name to denote `window`
5# we will use the terms `screen` and `window` interchangeably
6w = turtle.Screen()
7
8# let's set a title for the window
9w.title('Line')
10
11# t is an instance of Turtle
12# a Turtle is also known as a `pen`
13t = turtle.Turtle()
14
15# draw a line
16t.forward(50)
17
18# starts the event loop
19# the event loop listens for events
20turtle.done()
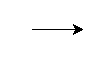
Line output.
17.2. Draw a square
Here’s a simple program to draw a square.
1import turtle
2
3# w is an instance of Screen
4# we choose w as the variable name to denote `window`
5# we will use the terms `screen` and `window` interchangeably
6w = turtle.Screen()
7
8# let's set a title for the window
9w.title('Square')
10
11# t is an instance of Turtle
12# a Turtle is also known as a `pen`
13t = turtle.Turtle()
14
15# we will loop 4 times
16# each time, we make the turtle move forward and turn right 90 degrees
17for i in range(4):
18 t.forward(50)
19 t.right(90)
20
21# starts the event loop
22# the event loop listens for events
23turtle.done()

Square output.
17.3. Draw stars
Here’s code to draw a star.
1import turtle
2
3w = turtle.Screen()
4w.title('Star')
5
6t = turtle.Turtle()
7
8for i in range(5):
9 t.forward(50)
10 t.right(144)
11
12turtle.done()

Star output.
Let’s draw a fancy star.
1import turtle
2
3w = turtle.Screen()
4w.title('Fancy Star')
5
6t = turtle.Turtle()
7
8for i in range(20):
9 t.forward(i * 10)
10 t.right(144)
11
12turtle.done()
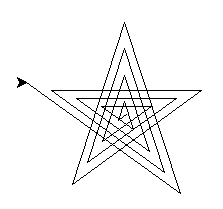
Star output.
17.4. Draw rectangular spirals
Let’s draw a rectangular spiral from the inside out. We will change the color that the turtle will use to red. We will also define the spiral
function to draw each spiral.
1import turtle
2
3def spiral(size, t, delta=-5):
4 for i in range(4):
5 t.fd(size)
6 t.left(90)
7 size = size + delta
8
9w = turtle.Screen()
10w.title('Inside Out')
11
12t = turtle.Turtle()
13t.color('red')
14nums = list(range(6, 147, 20))
15
16for num in nums:
17 spiral(num, t, delta=5)
18
19turtle.done()
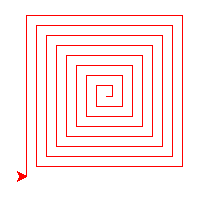
Inside-out rectangular spiral output.
Let’s draw a rectangular spiral from the outside-in. Since we are drawing the spirals from the outside-in, we will reverse the sizes.
1import turtle
2
3def spiral(size, t, delta=-5):
4 for i in range(4):
5 t.fd(size)
6 t.left(90)
7 size = size + delta
8
9w = turtle.Screen()
10w.title('Outside In')
11
12t = turtle.Turtle()
13
14t.color('blue')
15nums = list(range(26, 147, 20))
16nums.reverse()
17
18for num in nums:
19 spiral(num, t)
20
21turtle.done()
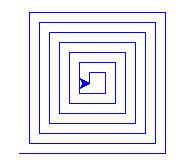
Outside-in rectangular spiral output.
17.5. Draw polygons
How about polygons? Let’s draw a hexagon.
1import turtle
2
3w = turtle.Screen()
4w.title('Hexagon')
5
6t = turtle.Turtle()
7
8n_sides = 6
9s_len = 70
10angle = 360.0 / n_sides
11
12for i in range(n_sides):
13 t.forward(s_len)
14 t.right(angle)
15
16turtle.done()
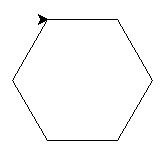
Hexagon output.
17.6. Draw a spiral helix
Let’s draw a spiral helix. First, let’s change the background color to black. Second, let’s define the colors that we want the pen to use. Lastly, since there’s a lot to draw, let’s set the speed of the pen to 100.
1import turtle
2
3w = turtle.Screen()
4w.title('Spiral Helix')
5w.bgcolor('black')
6
7colors = ['red', 'purple', 'blue', 'green', 'orange', 'yellow']
8
9t = turtle.Pen()
10t.speed(100)
11
12for x in range(360):
13 color = colors[x % len(colors)]
14 t.pencolor(color)
15 t.width(x / 100 + 1)
16 t.forward(x)
17 t.left(59)
18
19turtle.done()
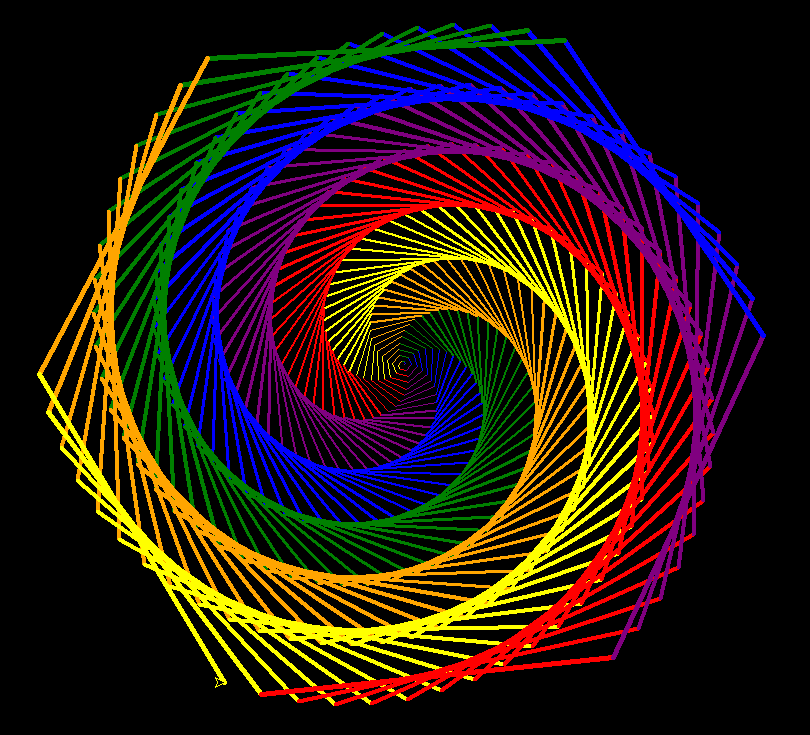
Spiral helix output.
17.7. Dots
Here’s an exercise in drawing dots. We use penup
to make sure no drawing is done when moving and dot
to place the pen down to create the dots. Additionally, if you want the change the shape of the pen from a classic arrow, you may use the shape
method. Below, for each row of dot we are drawing, we change the shape.
1import turtle
2
3t = turtle.Turtle()
4
5dot_distance = 25
6width = 5
7height = 7
8
9shapes = ['arrow', 'circle', 'classic', 'square', 'triangle', 'turtle']
10
11t.penup()
12
13for y in range(height):
14 shape = shapes[y % len(shapes)]
15
16 t.shape(shape)
17
18 for i in range(width):
19 t.dot()
20 t.forward(dot_distance)
21 t.backward(dot_distance * width)
22 t.right(90)
23 t.forward(dot_distance)
24 t.left(90)
25
26turtle.done()
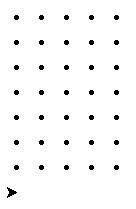
Dots output.
We may also stamp
out colorful patterns.
1import turtle
2
3t = turtle.Turtle()
4
5dot_distance = 25
6width = 5
7height = 7
8
9shapes = ['arrow', 'circle', 'classic', 'square', 'triangle', 'turtle']
10colors = ['red', 'purple', 'blue', 'green', 'orange', 'yellow']
11
12t.penup()
13
14for y in range(height):
15 shape = shapes[y % len(shapes)]
16 color = colors[y % len(colors)]
17
18 t.shape(shape)
19 t.pencolor(color)
20 t.color(color)
21
22 for i in range(width):
23 t.stamp()
24 t.forward(dot_distance)
25 t.backward(dot_distance * width)
26 t.right(90)
27 t.forward(dot_distance)
28 t.left(90)
29
30turtle.done()
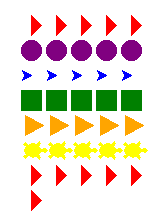
Stamp output.
17.8. Crazy lines
Let’s draw some crazy lines. We use penup
and pendown
to control when we want the pen to draw onto the canvas. Note that as we iterate through the angles, we speed up the pen’s drawing speed as well as change its colors.
1import turtle
2
3t = turtle.Turtle()
4
5colors = ['red', 'purple', 'blue', 'green', 'orange', 'yellow']
6speed = 10
7
8for i in range(180):
9 color = colors[i % 6]
10
11 t.pencolor(color)
12 t.speed(speed)
13
14 t.forward(100)
15 t.right(30)
16 t.forward(20)
17 t.left(60)
18 t.forward(50)
19 t.right(30)
20
21 t.penup()
22 t.setposition(0, 0)
23 t.pendown()
24
25 t.right(2)
26
27 speed = speed + 2
28
29turtle.done()
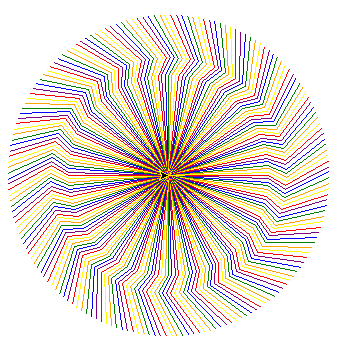
Crazy lines output.
17.9. Bright sun
Here’s a sun. Notice how we change the import statement from import turtle
to from turtle import *
? Now we have access to the begin_fill
and end_fill
methods, which fills up the shape we draw where we mark begin and end.
1from turtle import *
2
3speed(50)
4color('red', 'yellow')
5
6begin_fill()
7
8while True:
9 forward(200)
10 left(170)
11 if abs(pos()) < 1:
12 break
13
14end_fill()
15
16done()
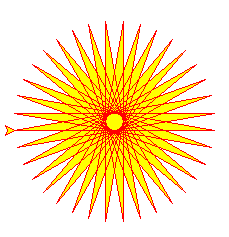
Sun output.
17.10. Events
We may also interact with the turtle through listening for events. In this example, we listen for key events using the screen’s onkey
function and call listen
after we have defined all the keys we are listening to. When a user presses
the up arrow, we move up
the down arrow, we move down
the left arrow, we move left
the right arrow, we move right
the
p
key, we toggle the pen up and down statethe
q
key, we quit the program
We use the pen’s setheading
to orient the cursor as appropriate (to the north, south, east or west) before we move.
1import turtle
2
3def up():
4 t.setheading(90)
5 t.forward(50)
6 state = 'up'
7 print(state)
8
9def down():
10 t.setheading(270)
11 t.forward(50)
12 state = 'down'
13 print(state)
14
15
16def right():
17 t.setheading(0)
18 t.forward(50)
19 state = 'right'
20 print(state)
21
22def left():
23 t.setheading(180)
24 t.forward(50)
25 state = 'left'
26 print(state)
27
28def toggle_pen_up_down():
29 if t.isdown():
30 t.penup()
31 print('pen up')
32 else:
33 t.pendown()
34 print('pen down')
35
36def close():
37 print('close')
38 turtle.bye()
39
40w = turtle.Screen()
41w.title('Event Listening')
42
43t = turtle.Turtle()
44p_state = 'down'
45
46w.onkey(up, 'Up')
47w.onkey(down, 'Down')
48w.onkey(right, 'Right')
49w.onkey(left, 'Left')
50w.onkey(close, 'q')
51w.onkey(toggle_pen_up_down, 'p')
52
53w.listen()
54
55turtle.done()
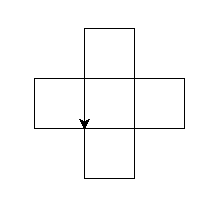
Event output.
17.11. Built-in examples
For even more examples, type in the following.
1python -m turtledemo